Demystifying Binary Search: An Algorithm for Quick Discovery
What is Binary Search?
Imagine you're rummaging through a phonebook, looking for a name that begins with "L". Most likely, you wouldn't start from the first page and flip through each one until you reach "L", right? A more plausible approach would be to open the phonebook around the middle and move to the left or right depending on where "L" lies.
Similarly, when searching for a word that starts with "P" in a dictionary, you'd probably start from the middle and navigate left or right, depending on the first letter of the word you're seeking.
Welcome to the world of binary search.
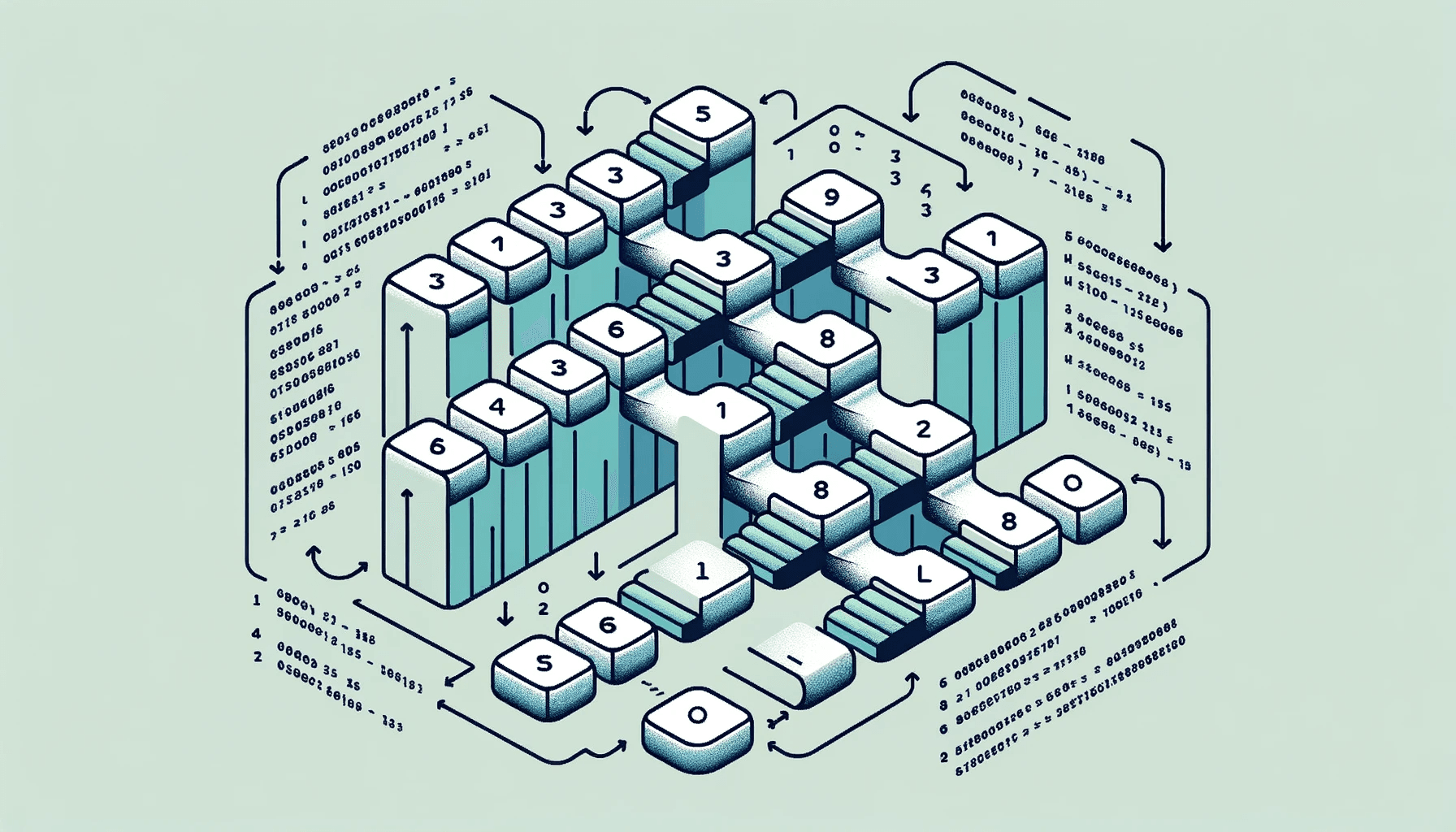
Understanding Binary Search
At the core, binary search is an algorithm designed to sniff out an element's location from a sorted list of elements. If the target element exists, the algorithm returns its location; if it doesn't, it returns None
(or -1
in some programming languages).
For instance, consider you're seeking a number between 1 and 100. To guess, you must take the fewest possible attempts. Throughout the process, I'll let you know if your guess is too high, too low, or right on the money.
A straightforward approach (known as linear search) would be to take a shot in the dark, starting from 1, then 2, then 3, so on and so forth. If my number happens to be 99, you'd need 99 attempts to guess correctly. Would you consider that efficient? Probably not.
However, if you kick off by guessing 50 (the middlemost value), I'd nudge you in the right direction – up or down. If my number is higher than 50, you can write off all numbers between 1 and 50 and look only between 51 and 100. And if it's lower, you ditch numbers between 51 and 100, focusing only on 1 to 49. By continually halving possibilities, you home in on my number using the binary search algorithm.
Here's a glimpse of how many numbers binary search eliminates in each round: 50 -> 25 -> 13 -> 7 -> 4 -> 2 -> 1. In just 7 steps, you'd hit the jackpot.
Now, apply this logic to a dictionary with 480,000 words. Binary search lets you find a word in, at most, 19 steps!
Broadly speaking, a binary search needs log₂ n steps to return the correct value, whereas linear search requires n
steps, where n
corresponds to the list's length.
In case you feel a slight frown due to the term 'logarithms', here's a refresher with an emphasis on how to compute exponentials. The expression log₁₀ 100 asks: "how many instances of 10 can we multiply to reach 100?". The answer is 2 because 10 * 10 = 100
. Therefore, log₁₀ 100 equals 2.
Note, however, that binary search works only if your list is sorted. What would ensue if the dictionary were not arranged in alphabetical order?
Execution Time
Let's go back to the linear search or a simple search where you check numbers sequentially. If you have a list of 100 numbers, worst-case scenario, you'd need 100 tries. For a list with 4 billion numbers, you'd need 4 billion attempts. The maximum tries are equivalent to the list's length, known as linear time.
Binary search differs. If your list has 100 items, you'd need at most 7 tries. If it contains 4 billion items, you need, at max, 32 tries. It's a potent algorithm, isn't it?
Implementation
Ready to see binary search in action? Here is a JavaScript implementation example to get you started:
function binarySearch(nums, item) {
let left = 0
let right = nums.length - 1
do {
let mid = Math.floor((left + right) / 2)
let guess = nums[mid]
if (guess === item) {
return mid
}
if (guess > item) {
// too high
right = mid - 1
} else {
// too low
left = mid + 1
}
} while (left <= right)
return -1
}
const result = binarySearch([1, 2, 3, 4, 5], 4) // 3
console.log({ result })
By running this code, you'll see that it successfully locates the number "4" at the third index in the array. That's the magic of binary search in action! Keep in mind, this is a simple JavaScript implementation. Depending on the layout or type of your data, you may need to adjust it to suit your scenario best.
In conclusion, binary search is an efficient algorithm that can save a lot of time and computing power when locating items in large, sorted datasets. It truly underscores the benefits of understanding your data and structuring it logically to optimize search operations. Happy coding, and may binary search be with you in your algorithmic journeys!