Is NodeJS single threaded or not?
There is a very common question in the NodeJS developer community about whether NodeJS is single-threaded. We first need to understand how NodeJS works underneath the scenes to find the answer.
Let's get started!
Getting to Know the Event Loop
The Event Loop is a built-in feature of NodeJS built around a cycle of events. This is how NodeJS executes all instructions in its algorithm and passes these instructions to the machine processor.
Event Loop is single-threaded. That is, all instructions are sent to a single thread, called the Event Queue, and then either each instruction in that list is processed in order, or they are handed off to another thread (Thread Pool) that will execute them in the background. This second thread that executes instructions in the background is made possible by libuv (a C library originally written for NodeJS to abstract non-blocking input/output operations).
As soon as each Event Loop operation is finished, NodeJS returns to the list of next tasks and focuses on executing the next non-blocking task.
Step by step
NodeJS is event-based (like requests, calls, etc). All these events are processed primarily in a single thread and may vary according to the type of operation (such as whether it is blocking I/O or not).
The step-by-step that NodeJS performs are:
- The operation arrives in the Event Queue;
- If it is the first operation in the list, the Event Loop consumes it immediately;
- The Event Loop checks whether this operation is fast or not to be executed;
- If it is a fast operation, it is processed and returns the answer so that the consumption of the next operations can be performed;
- If it is not a fast operation to be executed (such as operations involving filesystem, network, process, and other modules), the operation is sent to the Thread Pool so that the Event Loop is not blocked for too long;
- While the Thread Pool is executing longer tasks, the Event Loop keeps consuming the lightweight tasks from the Event Queue, so there is no blockage in the processing of the code;
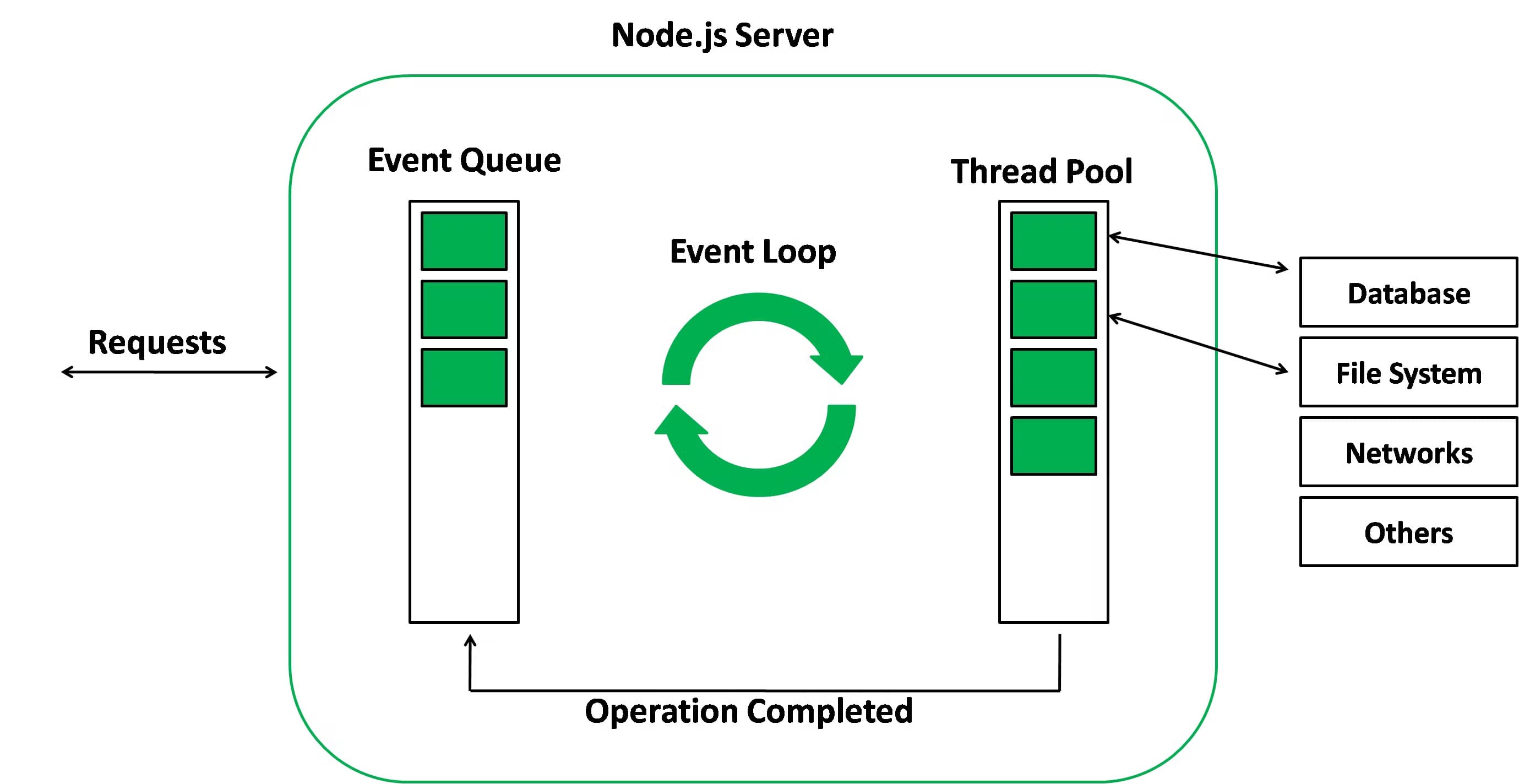
What makes NodeJS different from other languages?
The Thread Pool (where slower operations are executed in the background) has 4 threads by default, but this can be configured by hand to have varying threads. In other words, the Thread Pool is not single-threaded but is part of the NodeJS core.
The Thread Pool does not create a new thread for each operation but rather shares a pool of threads. This makes NodeJS more efficient concerning the consumption of computer resources. Because of this, NodeJS will use much less computational resources than an application made in PHP or .NET, for example (This does not mean that NodeJS is better or worse than PHP or .NET).
Here are some examples of how the NodeJS architecture works with single and multi-threaded operations
1 request on Google's website executes about 88 million instructions on the CPU. So NodeJS delegates to someone else (Thread Pool) to execute this instruction so that while this is happening in the background, NodeJS can execute another 88 million instructions in parallel through the Event Loop instead of blocking 100% of the operations.
Or when reading 1KB of data from a file, it takes 1.4 microseconds on an SSD that reads 200-730MB/s. On a 2GHz processor, this results in an execution of 28,000 instructions. Because of this, NodeJS does not block consumption and keeps this heavier operation in a background process.
Conclusion
NodeJS is single-threaded, but it relies on the help of the libuv library to perform some multi-threaded operations in the background.