Recursion Revealed: An Intuitive Guide for Software Engineers
Think about a Russian doll. You open the first doll to find a smaller doll inside, then another one in that, and this continues until you can't find any more dolls. This real-world scenario exhibits recursion, where a big problem (finding the smallest doll) can be solved by dividing it into smaller, simpler problems of the same type.
Recursion is pervasive in computer programming, enabling developers to tackle complex chaos with simple elegance. However, to some, it can appear daunting, or even unnecessary – when can't I just use a loop? I aim to dispel this unease by lending you my understanding of recursion, built especially for software engineering, with all of its beauty, drawbacks and common applications.
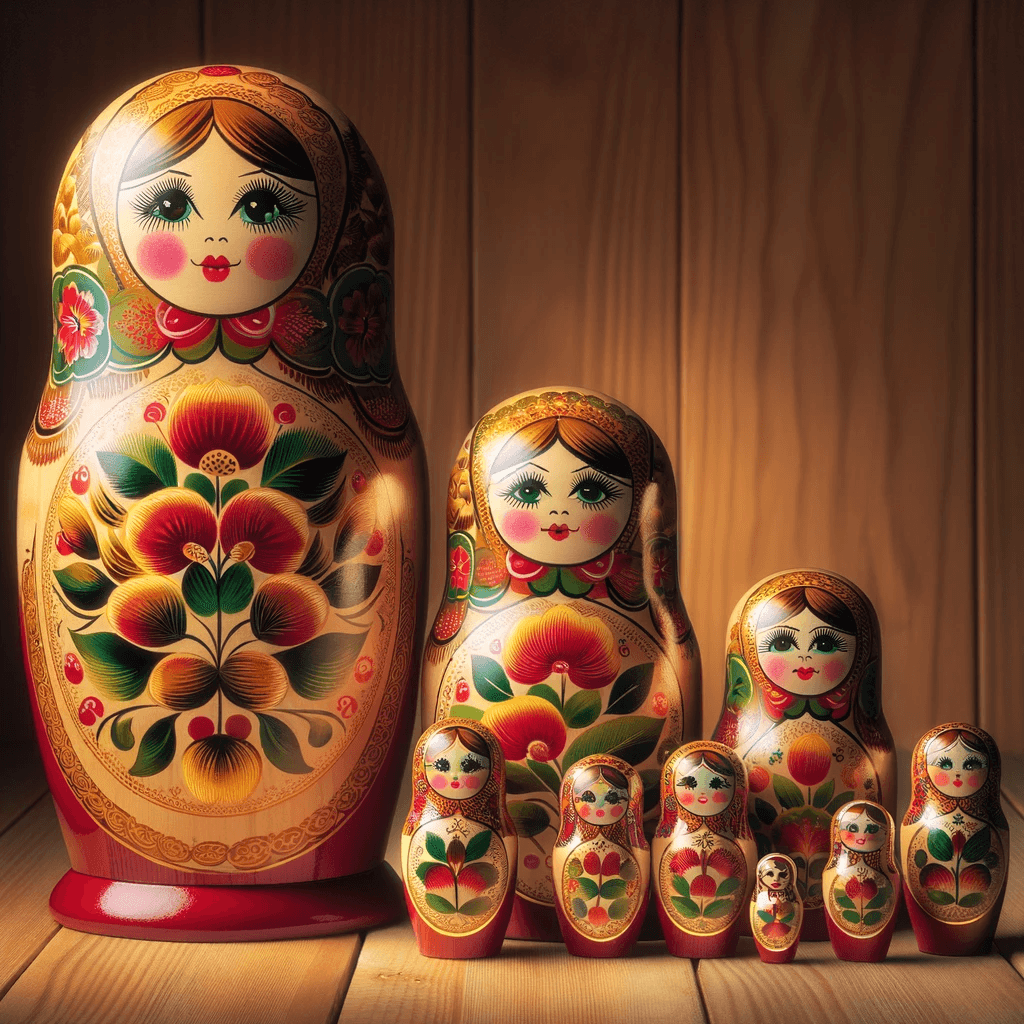
Spotting Recursion in everyday Scenarios
The suitcase conundrum: imagine you're rummaging through your basement and stumble upon a mysterious, locked suitcase. You suspect the key is hiding in one of many boxes lying around, but these boxes can further contain smaller boxes. A recursive solution to finding the key looks like this:
- Examine box contents.
- If another box lurks inside, go to step 1.
- If you find the key, celebrate!
On the other hand, you could set up a loop that throws all boxes on a pile, reviewing each until the key is found. While these strategies hit the same outcome, recursion undoubtedly adds an intuitive clarity to the answer's pathway.
Interestingly, this elegance does not translate into performance benefits. Sometimes, loops even have the upper hand when considering system resources.
Recursion Anatomy: Base Case and Recursive Case
A recursive function needs to know when to stop calling itself, ensuring we're safe from a dreaded infinite loop. Therefore, every recursive function has two essential parts:
- Base case: It defines the condition which stops the function from calling itself, thus avoiding an infinite loop.
- Recursive case: It specifies the condition under which the function calls itself, hence the recursion.
Call Stack Unwrapped
The 'stack' concept is integral to understanding recursion - and can be easily imagined by considering a pile of sticky notes. Each note could represent a task for, let's say, an upcoming BBQ party. You add tasks on top of the stack (push
), and you deal with them from the top too, reading and removing as you go (pop
) – that's a 'Stack'.
However, recursion deals with a specific version - 'Call Stack'. Every time a function is activated, a new frame is pushed onto the Call Stack. This Stack keeps track of the function to return to post the current function's completion. The concept extends to recursive functions, with every recursive call adding a new frame. The base case comes to rescue when all function calls have completed executing, popping frames off the Call Stack until it's empty.
Let's dissect this on a classic example: factorial function.
function factorial(x) {
if (x === 1) return 1
return x * factorial(x - 1)
}
The factorial
function calls itself to find the product of a number and the factorial of the one before it. When x
equals 1
, it stops the recursive calls, popping the frames and returning the computed value.
While the Call Stack aids recursion, overusing it might lead to memory issues. If you're saving numerous calls in memory (you've a very long stack), you could either rewrite your function to use loops instead of recursion or use tail recursion - a complex concept beyond the scope of this post, with limited support across programming languages.
More Meat on Recursion: Real-World Code Examples
Diving into Directories
A practical example of recursion is counting the total number of files in a directory and its sub-directories.
function countFiles(directory) {
let count = directory.files.length
for (const subDirectory of directory.subDirectories) {
count += countFiles(subDirectory)
}
return count
}
Adventuring in Array Summation
Arriving at simpler problems, consider summing an array of numbers through recursion.
function sum(array) {
if (array.length === 0) return 0
return array[0] + sum(array.slice(1))
}
The Famed Fibonacci Sequence
Computing the Fibonacci series is a classic recursion job - each number equals the sum of the two preceding ones.
function fibonacci(n) {
if (n <= 2) return 1
return fibonacci(n - 1) + fibonacci(n - 2)
}
console.log(fibonacci(7)) // Output: 13
Wrapping Up
So we've deciphered recursion. Gaining comfort with recursion can take a little time. Breaking up complex problems into smaller, similar problems - over and over again until a solution surfaces should become more natural with practice. It's a powerful tool that can effectively clear a lot of conceptual clutter, lend elegance to your solution and breed efficient code when used appropriately. So gear up for some recursive coding!