Deconstructing Problems with "Divide and Conquer" Technique
Have you ever faced a daunting task, like a jigsaw puzzle, and wondered where to start? Most of us would instinctively begin by sorting the pieces into small manageable groups, maybe by color or edge type. This illustrates a common problem-solving strategy, breaking a problem down into smaller parts to make it more manageable. In computer science, we use the same principle with a strategy known as "Divide and Conquer". In this article, we dive into the fundamentals of "Divide and Conquer" and show how it can be applied with recursive algorithms, using real-world examples.
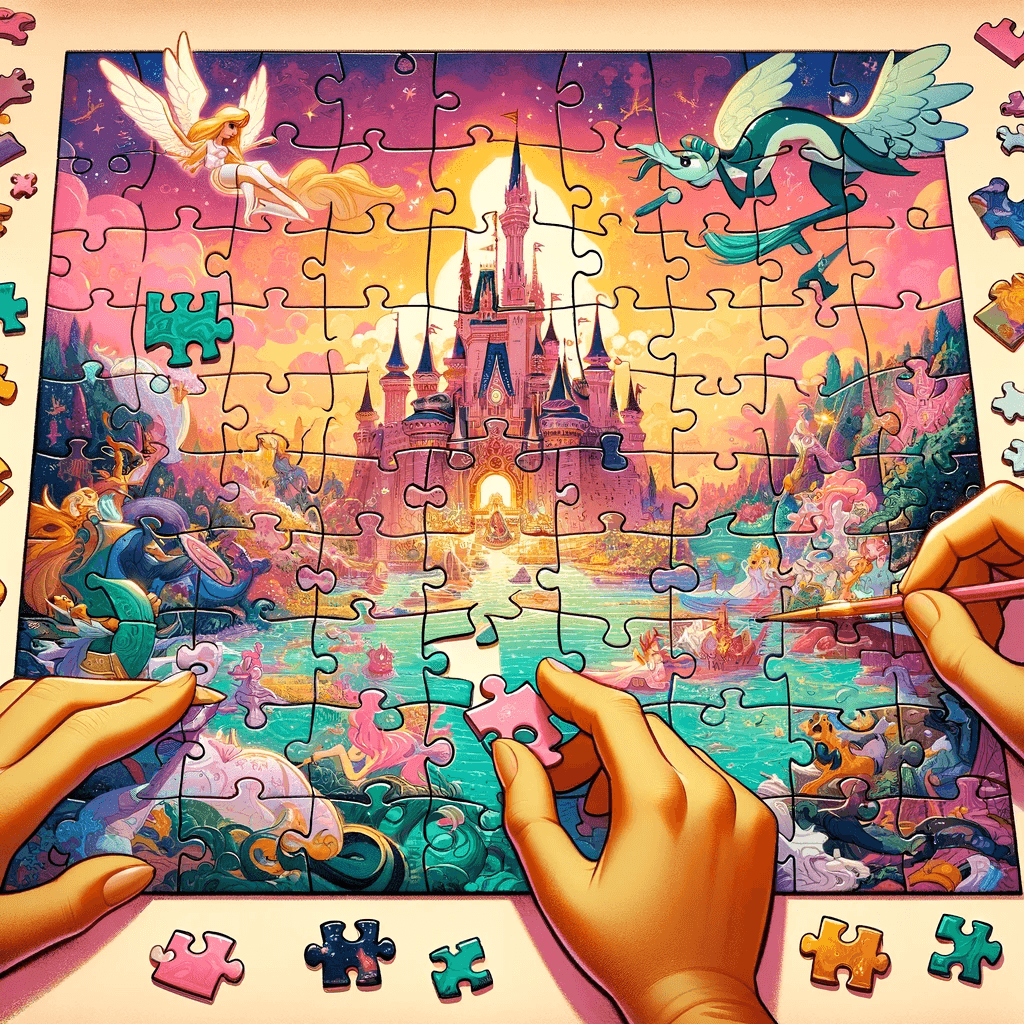
Unraveling Complexities with Divide and Conquer
"Divide and Conquer" is a popular problem-solving strategy in the field of computer science. It involves breaking down a complex problem into smaller subproblems. These subproblems are easier to solve, and their solutions can then be combined to address the original issue. Essentially, you're turning a big, complex problem into a series of more manageable tasks.
The key steps of the divide and conquer technique are:
- Identify the base case: This is the simplest possible instance of the problem.
- Divide or shrink your problem: With each iteration, you break down your problem until it matches the identified base case.
This technique forms the cornerstone of recursive algorithms, an important computer science concept I discussed in a previous article. Let's start applying these principles to our first problem.
Real-world Example: Dividing a Farm into Equal Squares
To bring this concept to life, let's consider a farmer who wishes to divide a plot of land, measuring 1680m by 640m, into equal square portions. The challenge here is to determine the maximum size of these square parcels.
Following the Divide and Conquer approach, our first step is to identify the base case. For this scenario, a simple base case would be if one of the sides were a multiple of the other. For instance, if we had a 50m by 25m plot, we could easily divide it into two squares each measuring 25m by 25m.
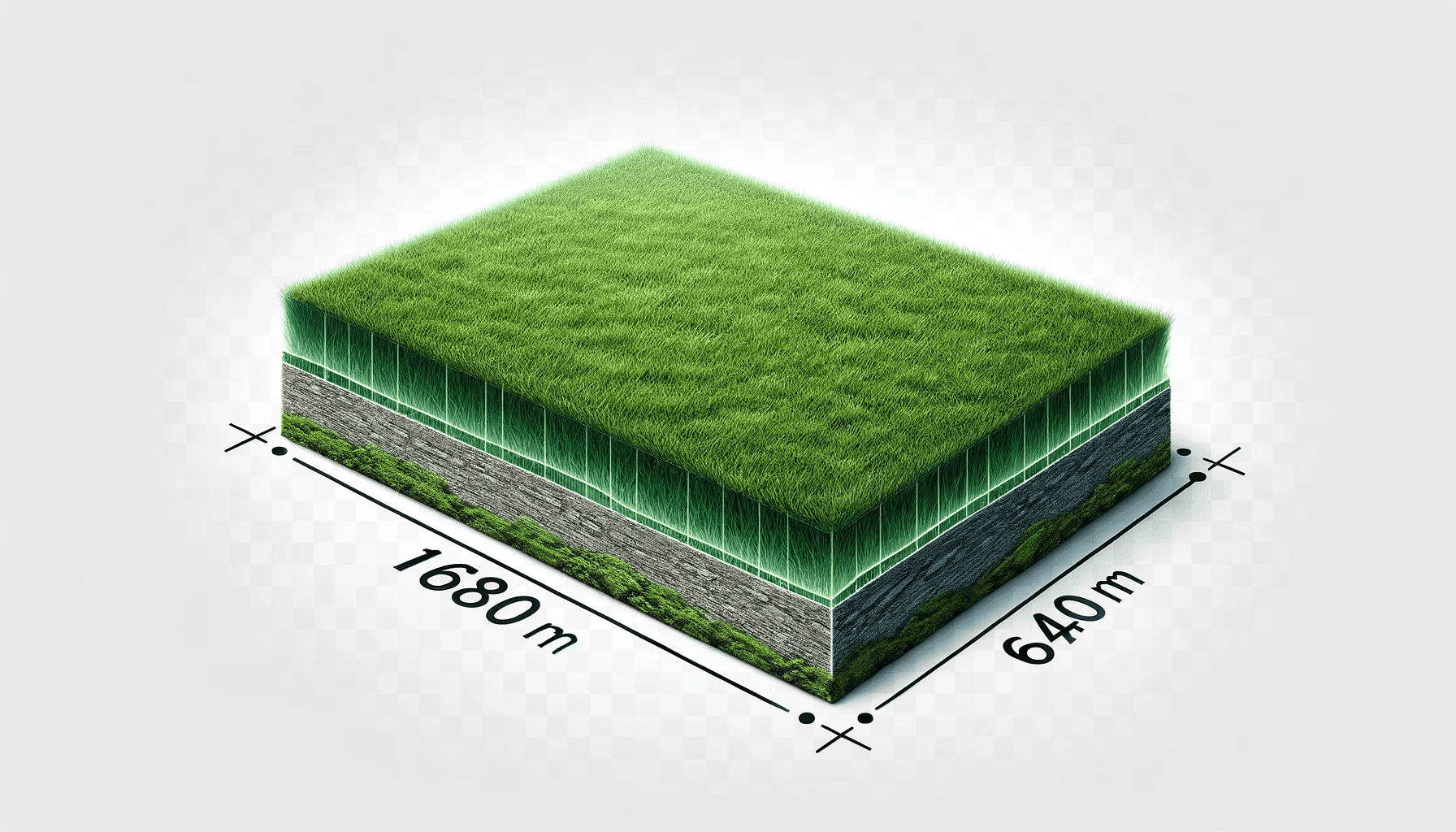
Now on to the next step: dividing the original problem until we encounter our base case. This is the point where Divide and Conquer really shines. Starting with the vast land area, we first identify the largest square that fits: in this case, a 640m x 640m square. We fit as many of these squares as possible into our area of land and then look at what's left over.
Following this process, we find that two 640m x 640m squares can fit within our plot, leaving behind a 400m x 640m area. From this point, we now need to divide this residual area in the same approach as before, shrinking our problem at every turn. The largest square that could fit in this remaining area is 400m x 400m which leaves a 240m x 400m area undivided.
Continuing this strategy, we fit in another square, this time 240m x 240m, leaving a 160m x 240m area. We then add a 160m square and are left with an area of 160m x 80m. At this point, we have reached our base case, as 160 is a multiple of 80. Dividing this last segment into squares leaves no segments unused. Therefore, for the original farmland, the largest square unit we can use to divide it into equal squares is 80m x 80m.
Putting it into Code: Summing Elements in an Array
This technique also has many practical applications in programming tasks. For instance, if you want to sum all the numbers in an array, you could easily execute this with a for loop:
function sum(arr) {
let total = 0
for (let i = 0; i < arr.length; i++) {
total += arr[i]
}
return total
}
However, this problem could also be solved recursively, using the Divide and Conquer technique. By defining our base case as an array with length 0 (or 1), we continuously reduce the size of the array with each recursive call until we reach the base case:
function sum(arr) {
if (arr.length === 0) {
// returns the base case
return 0
}
return arr[0] + sum(arr.slice(1)) // returns the sum of the first element and the sum of the rest of the array
}
Notice how the function reduces the problem size, brings it closer to the base case with each recursive call, and finally halts when it hits the base case. That is Divide and Conquer in action!
Further Practice: Dive Deeper with More Exercises
Further practice with the Divide and Conquer technique helps cement your understanding of recursive functions. Try your hand at the following exercises.
Recursive function to count the number of items in a list
function count(arr) {
if (arr.length === 0) {
// returns the base case
return 0
}
return 1 + count(arr.slice(1)) // returns 1 plus the count of the rest of the array
}
Recursive function to find the highest value in a list
function max(arr) {
if (arr.length === 1) {
// returns the base case
return arr[0]
}
return Math.max(arr[0], max(arr.slice(1))) // returns the highest value between the first element and the highest value of the rest of the array
}
Wrapping Up: Achieving Mastery in Divide and Conquer Strategy
In summary, the Divide and Conquer strategy allows us to tackle complex problems by breaking them down into more manageable subproblems. This technique then employs recursion to systematically solve these problems. Whether you're simplifying a land division problem or programming an array summing algorithm, this strategy greatly facilitates organized problem-solving.
Most importantly, this technique forms the foundation of various efficient sorting algorithms such as Quick Sort and Merge Sort, demonstrating its versatility and its critical role in computer science. Now that you've learned the fundamentals of "Divide and Conquer" techniques and their implementation in recursive algorithms, take some time to practice and solidify your understanding.
As with any skill, mastering these techniques comes with consistent practice. To guide your practice similar problems, I've included the code from this article and additional related examples in an open-source GitHub repository. Feel free to explore it at this link.
If you enjoyed this article and want even more great content like this, subscribe to my newsletter. Feel free to explore previous articles on recursion, selection sort, and other insightful subjects. Thanks for reading!